Leetcode 48. Rotate Image
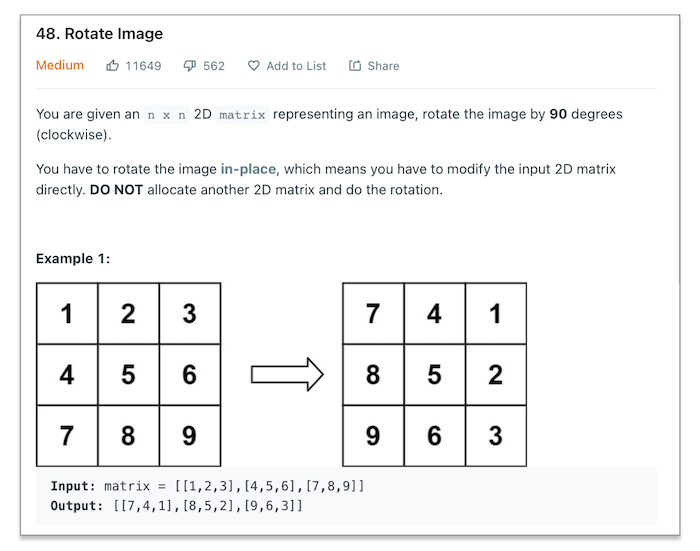
This question is frequently asked in interviews.
Solution
To visualize the rotation clockwise of 90 degress, let's focus on one column of the original matrix. As seen on the next figure the first column of the original matrix goes to the first row. Also the bottom element of the first column will go to the first place of the row.'
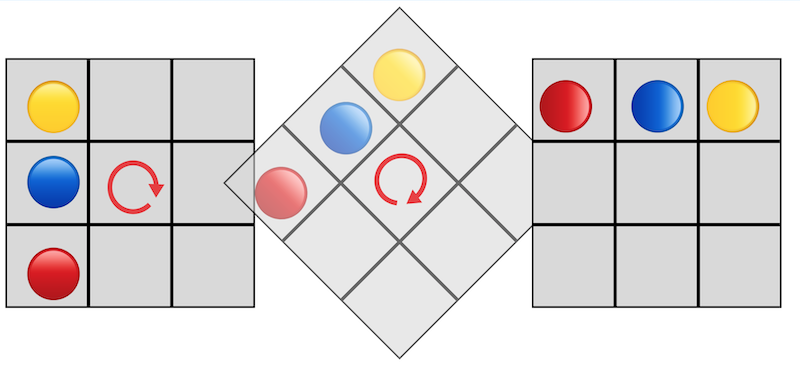
With this in mind, the solution is trivial:
class Solution {
func rotate(_ matrix: inout [[Int]]) {
let n = matrix.count
for i in matrix.indices {
var temp: [Int] = []
for j in stride(from: n - 1, through: 0, by: -1) {
temp.append(matrix[j][i])
}
matrix.append(temp)
}
matrix = matrix.suffix(matrix.count / 2)
}
}
Ther are other solutions, like transpose and reverse, like in the next figure:
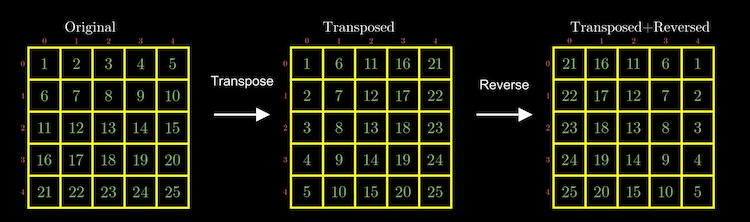
But as Swift does not have native functions for transpose, so it easier to present the first solution.